Android Toast: How to display toast message in android, toast in android, toast message in android, toast android studio, android toast example, toast in android studio, toast message example, toast.maketext() in android.
Hello Everyone,
If you are new to android and learning android basics and want to know how to show android toast or how to display android toast then you are in the right place. In this blog, I’m going to show you in detail a tutorial on toast in android studio or toast message in android studio, or toast in the android app.
What is Android Toast
Android toast provides simple feedback about an operation in a small popup. When showing toast messages, the current activity remains visible and interactive, which means it only fills the amount of space required for the message. Android Toasts automatically disappear after a timeout.
Definition by android.developer.com

Before diving into practicals you should know a few things about android toast
- Android Toast has two types of time durations. (Based on their time length)
- Short Toast
- Long Toast
- To show the toast in the android app, you should know the application context where you want to display the toast.
Why do we need Android Toast?
The reason is simple, it is used to display a message to the user about their actions in the app. So that they will know what they have done. It can be used as an informational text message or pop-up which gives some information to the user about their actions or the app functions. It is for a small period of time and a temporary message.
Can we change the position of a toast message?
By default, its position is at the bottom center of the screen. And yes we can change the position of the toast message anywhere on the screen. But it is only working in the older version of android, not in the latest one.
Let’s start
Android Toast: How to display toast message on android
Let’s have a look at the code which we need to show the toast in android
Toast.makeText(context, text, duration).show();
Overall Code which we need to make and show the Toast is:
Toast.makeText(getApplicationContext(),"Hello, This is Example of Toast Message in Android",Toast.LENGTH_SHORT).show();

A few steps that you need to follow are:
Step 1: Write down the Toast, it will import the class Toast.
Step 2: After Toast write down the method .makeText() it is a member of Toast class or we can say that it is a method that properly initializes the Toast Object.
This method takes three parameters.
- The application context.
- Text that should appear to the users.
- The duration that the toast should remain on the screen.
- it can be of two types: Toast.LENGTH_SHORT or Toast.LENGTH_LONG
Step 3: So, in the first parameter, we put the name of the activity with .this keyword on which we want to display the toast message. We can also use the getApplicationContext() method instead of naming specific activities.
Step 4: In the Second parameter, we provide the text that should appear to the users in String format.
Step 5: The third parameter will decide the length or duration of the time, whether it is short or long.
Step 6: Finally, we use the .show() method to display the android toast to users.
That’s all you need to do to show the toast message on android.
Let’s make a layout with one button, pressing it will show a toast message.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Toast" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
Now, place the toast message in a button click event listener, whenever we click that button it will show a toast message.
MainActivity.java
package com.startechcity.switchactivity; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; public class MainActivity extends AppCompatActivity { Button button; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); button = findViewById(R.id.button); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Toast.makeText(getApplicationContext(),"Hello, This is Example of Toast Message in Android",Toast.LENGTH_SHORT).show(); } }); } }
Output:
Showing the toast message at the default position in android.
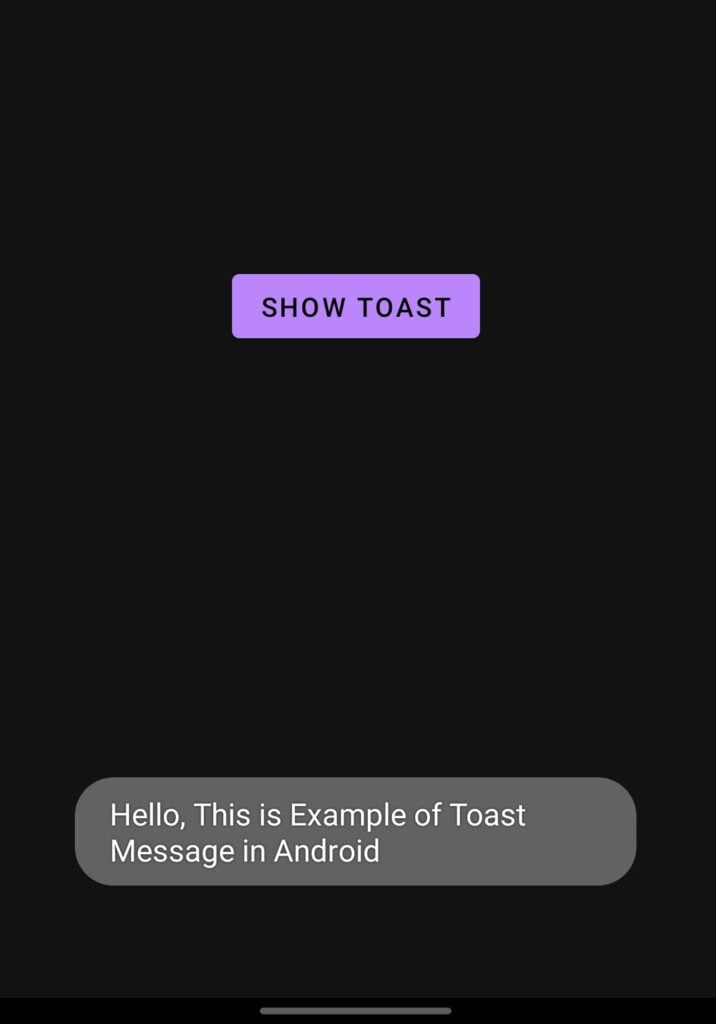
Support us by sharing it with others.